IDataObject
What is a Data Object?
Data Object is responsible for managing storage or data structures without containing any business logic. Unlike the Data Manager, which defines how an asset should behave, the Data Object focuses solely on handling the data itself, including encoding, decoding, and executing changes to the asset's state.
Data Objects are entrusted with managing transactions that affect the storage of Data Points. They can receive read()
and write()
requests from a Data Manager seeking access to a Data Point. Therefore, Data Objects operate based on a gating mechanism provided by a single Data Index.
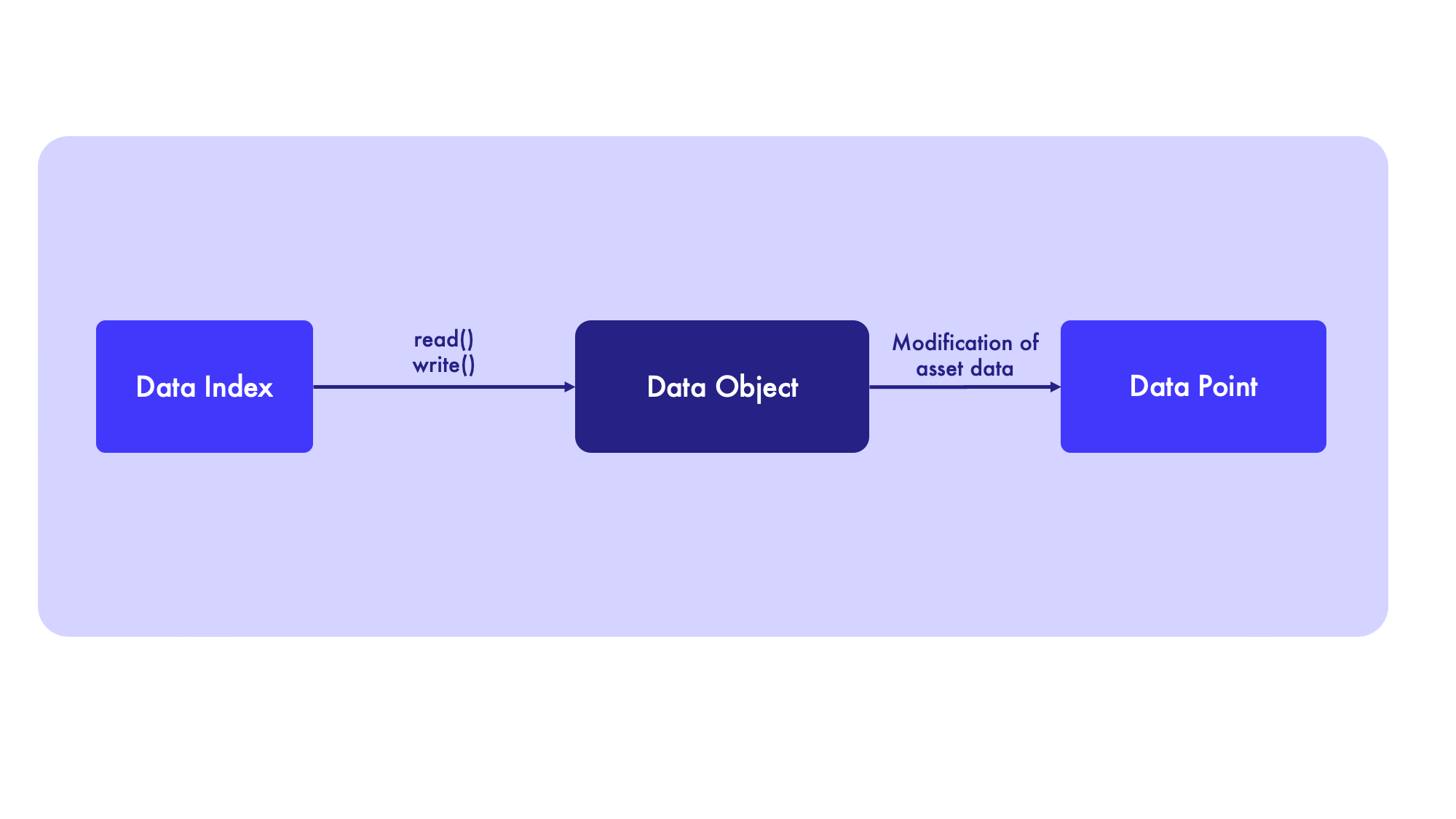
Fig 1. An operation coming to the Data Object from the Data Index modifies data structures associated with a Data Point.
Interface
When the Data Manager instructs the Data Index to execute an operation, the Data Object will handle the internal storage changes. It must inherit this interface.
Inside the Data Object, we should implement the intrinsic logic required to handle each write()
or read()
operation coming from a Data Manager. This logic should refer to the specific Data Point for each user or application, ensuring that only the targeted storage is modified.
At this point, the application of logic in the Data Manager or Data Index should look familiar:
//This method is defined at DataIndex
function write(address dobj, DataPoint dp, bytes4 operation, bytes calldata data) external onlyApprovedDM(dp) returns (bytes memory) {
return IDataObject(dobj).write(dp, operation, data);
}
Focus on the use of read()
and write()
operations.
//This method is defined at DataManager
function publicViewMethod1(address arg1, uint256 arg2, bytes argN) public view returns (bool) {
return
abi.decode(
dataObject.read(_datapoint, operation.selector, abi.encode(arg1, arg2, argN)),
(bool)
);
}
Methods
1. read()
function read(DataPoint dp, bytes4 operation, bytes calldata data) external view returns (bytes memory);
Description | Parameters | Returns | Modifiers |
---|---|---|---|
Reads stored data | dp : Identifier of the Data Pointoperation : Read operation to execute on the datadata : Operation-specific data | Operation-specific data | external view |
2. write()
function write(DataPoint dp, bytes4 operation, bytes calldata data) external returns (bytes memory);
Description | Parameters | Returns | Modifiers |
---|---|---|---|
Stores data | dp : Identifier of the Data Pointoperation : Write operation to execute on the datadata : Operation-specific data | Operation-specific data (can be empty) | external |
3. setDataIndexImplementation()
function setDataIndexImplementation(DataPoint dp, IDataIndex newImpl) external;
Description | Parameters | Returns | Modifiers |
---|---|---|---|
Sets Data Index Implementation | dp : Identifier of the Data PointnewImpl : Address of the new Data Index implementation | None | external |
More details of the storage management in Data Objects functions can be found in the Data Object Reference Implementation.